Resilient
Golem detects faults and transparently migrates running workers to new nodes without disruption or loss of state.
Robust
Golem automatically applies customizable retry policies to all recoverable failures, ending cloud flakiness once and for all.
Serverless
Golem lets you focus on business logic, and uses standards like OpenAPI to create APIs for your workers automatically.
Stateful
Golem automatically preserves worker state between requests, regardless of failures, reducing the need for databases.
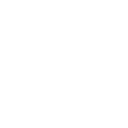
Transactional
Golem brings transactions to your business logic, guaranteeing exactly-once semantics, even in the presence of failures.
Composable
Golem brings compositionality to serverless through the WASM component model, enabling polyglot reuse.
.png)
Scalable
Golem automatically scales different components of your application to minimize infrastructure costs and maximize uptime.
Observable*
Golem provides built-in OTel metrics and tracing to hook into observability platforms so you can understand your applications.
Secure
Golem sandboxes and locks down every worker, providing them with capabilities and validated input, eliminating common vulnerabilities.
*Coming Soon